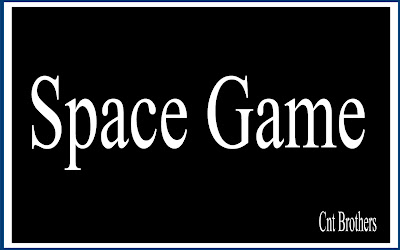
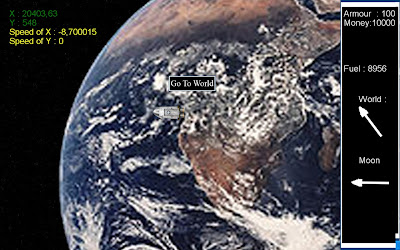
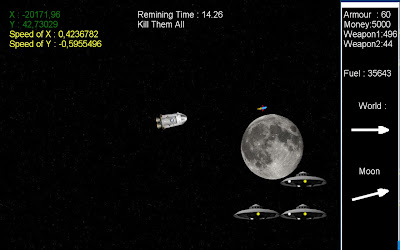
This is the 2D game that i made in XNA,
I spent my 1 week to make it.
It is not a big dial, i hope you like it.
Download Space Game
CODS OF SPACE GAME
GAME 1
using System;
using System.Collections.Generic;
using System.Linq;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Audio;
using Microsoft.Xna.Framework.Content;
using Microsoft.Xna.Framework.GamerServices;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Input;
using Microsoft.Xna.Framework.Media;
using Microsoft.Xna.Framework.Net;
using Microsoft.Xna.Framework.Storage;
using System.IO;
using System.Text;
namespace Space_Game
{
public class Game1 : Microsoft.Xna.Framework.Game
{
GraphicsDeviceManager graphics;
SpriteBatch spriteBatch;
Texture2D cntbrothers;
int sayim = 0;
Texture2D menu;
SpriteFont yazi;
bool oyunabasla = false;
int oyunabaslassayim = 0;
bool creditssayimcanli = false;
int creditsssayim = 0;
Texture2D control;
SoundEffect ses;
SoundEffect ses2;
#region fontlar
SpriteFont velocityX;
SpriteFont velocityY;
SpriteFont pozX;
SpriteFont pozY;
SpriteFont yakitfont;
SpriteFont kalanates1yazisi;
SpriteFont kalanates2yazisi;
SpriteFont sicagadayanmagucuyazisi;
SpriteFont sogugadayanmagucuyazisi;
SpriteFont zirhgucuyazisi;
SpriteFont koruyucukalkanyazisi;
SpriteFont hizlanmagucuyazisi;
SpriteFont hizgucuyazisi;
SpriteFont donushiziyazisi;
SpriteFont durusgucuyazisi;
SpriteFont durusgucusuperyazisi;
SpriteFont aciklayiciyazi;
SpriteFont parayazisi;
#endregion
#region baslangic kodlari
const int ScreenWidth = 1280;
const int ScreenHeight = 830;
Texture2D Arac;
Texture2D backgroundTexture;
Vector2 Merkez;
Vector2 Pozisyon = new Vector2(22000, 548);
Vector2 Hiz;
float Rota;
Vector2 cameraPosition;
KeyboardState Tusabas;
Texture2D agirliknoktasi;
Vector2 agirliknoktasipozisyon;
#endregion
#region ufo1
//Texture2D ufo1;
//Vector2 ufo1pozisyon = new Vector2(650, 300);
//Vector2 ufo1merkez;
//Vector2 ufo1hiz;
//float ufo1rota;
#endregion
#region gezegenler
Texture2D ay;
Vector2 aypozisyon = new Vector2(-20000, 0);
Vector2 ayhiz;
float ayrota;
Vector2 aymerkez;
Texture2D dunya;
Vector2 dunyapozisyon = new Vector2(+20000, 0);
Vector2 dunyahiz;
float dunyarota;
Vector2 dunyamerkez;
Texture2D venus;
Vector2 venuspozisyon = new Vector2(-150000, 0);
Vector2 venushiz;
float venusrota;
Vector2 venusmerkez;
Texture2D mars;
Vector2 marspozisyon = new Vector2(+150000, 0);
Vector2 marshiz;
float marsrota;
Vector2 marsmerkez;
#endregion
#region ates1
const int maxates1 = 500;
Game2[] ates1;
int ates1sayim = 0;
bool ates1canlilik = false;
bool ates1canlilik1 = false;
bool ates1bizeetkisi = false;
int kalanates1sayisi;
#endregion
#region ates2
const int maxates2 = 50;
Game2[] ates2;
int ates2sayim = 0;
bool ates2canlilik = false;
bool ates2canlilik2 = false;
int kalanates2sayisi;
#endregion
#region arac ozellikleri
int yakitdegeri = 10000;
float zirhgucu = 100;
int hizgucu = 50;
float hizlanmagucu = 0.5f;
float donushizi = 0.5f;
int durusgucu = 2;
int para = 10000;
int olum;
#endregion
#region bi kere allar
int bikerealzirhgucu = 0;
int bikerealhiz = 0;
int bikerealhizlanma = 0;
int bikerealdurus = 0;
int bikerealdonus = 0;
int bikerealsicak = 0;
int bikerealsoguk = 0;
int bikerealkoruyucukalkan = 0;
int bikerealdahahizli = 0;
int bikerealyerbulucu = 1;
int bikerealsuperfren = 0;
int bikerealgorunmezlik = 0;
int bikerealrobot216 = 0;
int bikerealimhaetme = 0;
int bikerealzirh = 0;
int bikerealyakit = 0;
int bikerealsilah2 = 0;
int bikerealsilah3 = 0;
int bikerealsilah4 = 0;
#endregion
#region karisik
Texture2D goreval;
Texture2D tukenenler;
Texture2D panel1;
Texture2D yanyuz;
Texture2D dunyayain;
Texture2D havalan;
Texture2D geridon;
int tikla1tikla2 = -1;
int butondegiskeni;
#endregion
#region araci gelistir
Texture2D hizgucugelistir;
Texture2D yerbulucual;
Texture2D yerbulucu;
Vector2 yerbulucuhiz;
Vector2 yerbulucumerkez;
Vector2 yerbulucupozisyon;
float yerbulucurota;
Texture2D yerbulucuok1;
Texture2D yerbulucuok2;
float yerbulucuokrota;
float yerbulucuokrota2;
Vector2 yerbulucuokmerkez;
Vector2 hedefpozisyon;
SpriteFont kalanyolyazisi;
float kalanyolX;
float kalanyolY;
float kalanyolXkalanyolY;
#endregion
#region tukenenler
Texture2D zirh;
Texture2D yakit;
Texture2D silah2;
Texture2D silah3;
#endregion
#region goktaslari
Texture2D goktasi1;
Vector2 goktasi1pozisyon = new Vector2(400, 400);
Vector2 goktasi1merkez;
Vector2 goktasi1hiz;
float goktasi1rota;
Texture2D goktasi2;
Vector2 goktasi2pozisyon = new Vector2(15000, 400);
Vector2 goktasi2merkez;
Vector2 goktasi2hiz;
float goktasi2rota;
Texture2D goktasi3;
Vector2 goktasi3pozisyon = new Vector2(25000, 400);
Vector2 goktasi3merkez;
Vector2 goktasi3hiz;
float goktasi3rota;
Texture2D goktasi4;
Vector2 goktasi4pozisyon = new Vector2(30000, 400);
Vector2 goktasi4merkez;
Vector2 goktasi4hiz;
float goktasi4rota;
#endregion
#region gorev1
SpriteFont gorev1yazisi1;
SpriteFont gorev1yazisi2;
SpriteFont gorev1yazisi3;
int gorev1stepleri = 0;
Texture2D uydu;
SpriteFont gorev1zamanyazisi;
int gorev1zamandakika = 05;
int gorev1zamansaniye = 00;
int gorev1zamansalise = 100;
int gorev1bitiris = 0;
#endregion
#region gorev2
int gorev2stepleri = 0;
Texture2D gorev2ufo;
int ufo1can = 100;
int ufo2can = 100;
int ufo3can = 100;
int ufo4can = 100;
Texture2D gorev2agirliknoktasi;
Vector2 gorev2agirliknoktasipozisyonu;
float ufo1rota;
float ufo1atesrota;
float ufo2rota;
float ufo2atesrota;
float ufo3rota;
float ufo3atesrota;
float ufo4rota;
float ufo4atesrota;
Vector2 ufo1merkez;
Texture2D ufo1ates;
Vector2 ufo1atespozisyon = new Vector2(-19950, -250);
int ufo1atessayim;
Vector2 ufo1ateshiz;
Vector2 ufo2merkez;
Texture2D ufo2ates;
Vector2 ufo2atespozisyon = new Vector2(-19950, -150);
int ufo2atessayim;
Vector2 ufo2ateshiz;
Vector2 ufo3merkez;
Texture2D ufo3ates;
Vector2 ufo3atespozisyon = new Vector2(-19950, 200);
int ufo3atessayim;
Vector2 ufo3ateshiz;
Vector2 ufo4merkez;
Texture2D ufo4ates;
Vector2 ufo4atespozisyon = new Vector2(-19950, 300);
int ufo4atessayim;
Vector2 ufo4ateshiz;
int ufo5can = 100;
int ufo6can = 100;
int ufo7can = 100;
int ufo8can = 100;
float ufo5rota;
float ufo5atesrota;
float ufo6rota;
float ufo6atesrota;
float ufo7rota;
float ufo7atesrota;
float ufo8rota;
float ufo8atesrota;
Vector2 ufo5merkez;
Texture2D ufo5ates;
Vector2 ufo5atespozisyon = new Vector2(-19850, -250);
int ufo5atessayim;
Vector2 ufo5ateshiz;
Vector2 ufo6merkez;
Texture2D ufo6ates;
Vector2 ufo6atespozisyon = new Vector2(-19850, -150);
int ufo6atessayim;
Vector2 ufo6ateshiz;
Vector2 ufo7merkez;
Texture2D ufo7ates;
Vector2 ufo7atespozisyon = new Vector2(-19850, 200);
int ufo7atessayim;
Vector2 ufo7ateshiz;
Vector2 ufo8merkez;
Texture2D ufo8ates;
Vector2 ufo8atespozisyon = new Vector2(-19850, 300);
int ufo8atessayim;
Vector2 ufo8ateshiz;
int ufo9can = 100;
int ufo10can = 100;
int ufo11can = 100;
int ufo12can = 100;
float ufo9rota;
float ufo9atesrota;
float ufo10rota;
float ufo10atesrota;
float ufo11rota;
float ufo11atesrota;
float ufo12rota;
float ufo12atesrota;
Vector2 ufo9merkez;
Texture2D ufo9ates;
Vector2 ufo9atespozisyon = new Vector2(-19550, -250);
int ufo9atessayim;
Vector2 ufo9ateshiz;
Vector2 ufo10merkez;
Texture2D ufo10ates;
Vector2 ufo10atespozisyon = new Vector2(-19550, -150);
int ufo10atessayim;
Vector2 ufo10ateshiz;
Vector2 ufo11merkez;
Texture2D ufo11ates;
Vector2 ufo11atespozisyon = new Vector2(-19550, 200);
int ufo11atessayim;
Vector2 ufo11ateshiz;
Vector2 ufo12merkez;
Texture2D ufo12ates;
Vector2 ufo12atespozisyon = new Vector2(-19550, 300);
int ufo12atessayim;
Vector2 ufo12ateshiz;
#endregion
public Game1()
{
Content.RootDirectory = "Content";
graphics = new GraphicsDeviceManager(this);
graphics.PreferredBackBufferWidth = ScreenWidth;
graphics.PreferredBackBufferHeight = ScreenHeight;
}
protected override void Initialize()
{
base.Initialize();
}
protected override void LoadContent()
{
spriteBatch = new SpriteBatch(GraphicsDevice);
cntbrothers = Content.Load
menu = Content.Load
yazi = Content.Load
control = Content.Load
ses = Content.Load
ses2 = Content.Load
#region baslangic
backgroundTexture = Content.Load
if (zirhgucu > 0)
{
Arac = Content.Load
}
agirliknoktasi = Content.Load
#endregion
#region fonts
velocityX = Content.Load
velocityY = Content.Load
pozX = Content.Load
pozY = Content.Load
yakitfont = Content.Load
kalanates1yazisi = Content.Load
kalanates2yazisi = Content.Load
sicagadayanmagucuyazisi = Content.Load
sogugadayanmagucuyazisi = Content.Load
koruyucukalkanyazisi = Content.Load
zirhgucuyazisi = Content.Load
hizlanmagucuyazisi = Content.Load
hizgucuyazisi = Content.Load
donushiziyazisi = Content.Load
durusgucuyazisi = Content.Load
durusgucusuperyazisi = Content.Load
aciklayiciyazi = Content.Load
parayazisi = Content.Load
kalanyolyazisi = Content.Load
#endregion
#region ufo1
//ufo1 = Content.Load
#endregion
#region gezegenler
ay = Content.Load
dunya = Content.Load
venus = Content.Load
mars = Content.Load
#endregion
#region ates1
ates1 = new Game2[maxates1];
for (int i = 0; i < maxates1; i++)
{
ates1[i] = new Game2(Content.Load
"gorev2\\ates2"));
kalanates1sayisi = i + 1;
}
#endregion
#region ates2
ates2 = new Game2[maxates2];
for (int i = 0; i < maxates2; i++)
{
ates2[i] = new Game2(Content.Load
"Resimler\\ates1"));
kalanates2sayisi = i + 1;
}
#endregion
#region karisik
goreval = Content.Load
tukenenler = Content.Load
panel1 = Content.Load
yanyuz = Content.Load
dunyayain = Content.Load
geridon = Content.Load
#endregion
#region tukenenler
zirh = Content.Load
yakit = Content.Load
silah2 = Content.Load
silah3 = Content.Load
hizgucugelistir = Content.Load
yerbulucual = Content.Load
yerbulucuok1 = Content.Load
yerbulucuok2 = Content.Load
#endregion
#region gok taslari
goktasi1 = Content.Load
goktasi2 = Content.Load
goktasi3 = Content.Load
goktasi4 = Content.Load
#endregion
#region gorev1
gorev1yazisi1 = Content.Load
gorev1yazisi2 = Content.Load
gorev1yazisi3 = Content.Load
uydu = Content.Load
gorev1zamanyazisi = Content.Load
#endregion
#region gorev2
gorev2ufo = Content.Load
ufo1ates = Content.Load
gorev2agirliknoktasi = Content.Load
ufo2ates = Content.Load
ufo3ates = Content.Load
ufo4ates = Content.Load
gorev2ufo = Content.Load
ufo5ates = Content.Load
gorev2agirliknoktasi = Content.Load
ufo6ates = Content.Load
ufo7ates = Content.Load
ufo8ates = Content.Load
ufo9ates = Content.Load
ufo10ates = Content.Load
ufo11ates = Content.Load
ufo12ates = Content.Load
#endregion
}
protected override void UnloadContent()
{
}
protected override void Update(GameTime gameTime)
{
if (Tusabas.IsKeyDown(Keys.Escape))
{
this.Exit();
}
HandleInput();
UpdateCamera();
UpdateCat();
UpdateUfo1();
Updateates1();
Updateates2();
Updateates1sayim();
Updateates2sayim();
Updateyerbulucuok();
UpdateGorev2ufo();
base.Update(gameTime);
}
void UpdateCat()
{
Merkez = new Vector2(Arac.Width / 2, Arac.Height / 2);
ufo1merkez = new Vector2(gorev2ufo.Width / 2, gorev2ufo.Height / 2);
Pozisyon += Hiz;
yerbulucurota += 0.1f;
yerbulucuokmerkez = new Vector2(yerbulucuok1.Width / 2, yerbulucuok1.Height / 2);
yerbulucuokmerkez = new Vector2(yerbulucuok2.Width / 2, yerbulucuok2.Height / 2);
if (Hiz.X <= -hizgucu)
{
Hiz.X = -hizgucu;
}
if (Hiz.Y <= -hizgucu)
{
Hiz.Y = -hizgucu;
}
if (Hiz.X >= hizgucu)
{
Hiz.X = hizgucu;
}
if (Hiz.Y >= hizgucu)
{
Hiz.Y = hizgucu;
}
if (yakitdegeri > 0)
{
//YÖN TUŞLARI
if (Tusabas.IsKeyDown(Keys.Left) && butondegiskeni != 0)
{
if (Hiz.X <= -4)
{
Hiz.X += 0.04f;
if (Hiz.X == 0)
{
Hiz.X = 0;
}
}
if (Hiz.Y <= -4)
{
Hiz.Y += 0.04f;
if (Hiz.Y == 0)
{
Hiz.Y = 0;
}
}
if (Hiz.X >= +4)
{
Hiz.X -= 0.04f;
if (Hiz.X == 0)
{
Hiz.X = 0;
}
}
if (Hiz.Y >= +4)
{
Hiz.Y -= 0.04f;
if (Hiz.Y == 0)
{
Hiz.Y = 0;
}
}
Rota -= donushizi / 100f;
//robot216rota = Rota;
foreach (Game2 icin in ates1)
{
if (icin.ruh == false)
{
icin.rotation -= donushizi / 100f;
}
}
foreach (Game2 icin in ates2)
{
if (icin.ruh == false)
{
icin.rotation -= donushizi / 100f;
}
}
yakitdegeri = yakitdegeri - 2;
}
if (Tusabas.IsKeyDown(Keys.Right) && butondegiskeni != 0)
{
if (Hiz.X <= -4)
{
Hiz.X += 0.04f;
if (Hiz.X == 0)
{
Hiz.X = 0;
}
}
if (Hiz.Y <= -4)
{
Hiz.Y += 0.04f;
if (Hiz.Y == 0)
{
Hiz.Y = 0;
}
}
if (Hiz.X >= +4)
{
Hiz.X -= 0.04f;
if (Hiz.X == 0)
{
Hiz.X = 0;
}
}
if (Hiz.Y >= +4)
{
Hiz.Y -= 0.04f;
if (Hiz.Y == 0)
{
Hiz.Y = 0;
}
}
Rota += donushizi / 100f;
//robot216rota = Rota;
foreach (Game2 icin in ates1)
{
if (icin.ruh == false)
{
icin.rotation += donushizi / 100f;
}
}
foreach (Game2 icin in ates2)
{
if (icin.ruh == false)
{
icin.rotation += donushizi / 100f;
}
}
yakitdegeri = yakitdegeri - 2;
}
if (Tusabas.IsKeyDown(Keys.Up) && butondegiskeni != 0)
{
Hiz -= new Vector2(
(float)Math.Cos(Rota),
(float)Math.Sin(Rota)) * hizlanmagucu / 10f;
yakitdegeri = yakitdegeri - 6;
}
if (Tusabas.IsKeyDown(Keys.Down) && butondegiskeni != 0)
{
if (Hiz.X >= 1)
{
Hiz.X -= durusgucu / 10f;
if (Hiz.X <= 0)
{
Hiz.X = 0;
}
}
if (Hiz.X <= -1)
{
Hiz.X += durusgucu / 10f;
if (Hiz.X >= 0)
{
Hiz.X = 0;
}
}
if (Hiz.Y >= 1)
{
Hiz.Y -= durusgucu / 10f;
if (Hiz.Y <= 0)
{
Hiz.Y = 0;
}
}
if (Hiz.Y <= -1)
{
Hiz.Y += durusgucu / 10f;
if (Hiz.Y >= 0)
{
Hiz.Y = 0;
}
}
if (Hiz.X > 1 || Hiz.X < -1 || Hiz.Y > 1 || Hiz.Y < -1)
{
yakitdegeri = yakitdegeri - 1;
}
}
//INTERSECT INTERSECT INTERSECT INTERSECT INTERSECT
Rectangle ayrect = new Rectangle(
(int)aypozisyon.X,
(int)aypozisyon.Y,
ay.Width * 3,
ay.Height * 3);
Rectangle venusrect = new Rectangle(
(int)venuspozisyon.X,
(int)venuspozisyon.Y,
venus.Width,
venus.Height);
Rectangle dunyarect = new Rectangle(
(int)dunyapozisyon.X,
(int)dunyapozisyon.Y,
dunya.Width,
dunya.Height);
Rectangle marsrect = new Rectangle(
(int)marspozisyon.X,
(int)marspozisyon.Y,
mars.Width,
mars.Height);
Rectangle aracrect = new Rectangle(
(int)Pozisyon.X,
(int)Pozisyon.Y + 25,
Arac.Width,
Arac.Height);
foreach (Game2 icin in ates1)
{
Rectangle ates1rect = new Rectangle(
(int)icin.position.X,
(int)icin.position.Y,
icin.nesne.Width,
icin.nesne.Height);
if (ates1rect.Intersects(aracrect) && ates1bizeetkisi == true)
{
}
}
}
}
void UpdateCamera()
{
Vector2 maxScroll = new Vector2(ScreenWidth, ScreenHeight) / 100;
const float catSafeArea = 0.7f;
maxScroll *= catSafeArea;
maxScroll -= new Vector2(Arac.Width, Arac.Height) / 2;
Vector2 min = Pozisyon - maxScroll;
Vector2 max = Pozisyon + maxScroll;
cameraPosition.X = MathHelper.Clamp(cameraPosition.X, min.X, max.X);
cameraPosition.Y = MathHelper.Clamp(cameraPosition.Y, min.Y, max.Y);
}
void UpdateUfo1()
{
//ufo1merkez = new Vector2(ufo1.Height, ufo1.Width) / 2;
}
#region update atesler
void Updateates1()
{
foreach (Game2 icin in ates1)
{
icin.position += -icin.velocity;
}
if (Tusabas.IsKeyDown(Keys.A) && ates1canlilik == true && ates1canlilik1 == true)
{
atesleme1();
ates1canlilik = false;
ates1bizeetkisi = false;
}
}
void Updateates1sayim()
{
if (ates1canlilik == false)
{
ates1sayim++;
}
if (ates1sayim >= 50)
{
ates1sayim = 0;
ates1bizeetkisi = true;
ates1canlilik = true;
}
}
#endregion
public void atesleme1()
{
foreach (Game2 icin in ates1)
{
//!icin.ruh demek icin == false demektir.
if (!icin.ruh)
{
kalanates1sayisi = kalanates1sayisi - 1;
icin.ruh = true;
icin.position = Pozisyon - icin.center;
icin.velocity = new Vector2(
(float)Math.Cos(Rota),
(float)Math.Sin(Rota)) * 30.0f - Hiz;
ses.Play();
return;
}
}
}
void Updateates2()
{
foreach (Game2 icin in ates2)
{
icin.position += -icin.velocity;
}
if (Tusabas.IsKeyDown(Keys.S) && ates2canlilik == true && ates2canlilik2 == true)
{
atesleme2();
ates2canlilik = false;
}
}
void Updateates2sayim()
{
if (ates2canlilik == false)
{
ates2sayim++;
}
if (ates2sayim >= 200)
{
ates2sayim = 0;
ates2canlilik = true;
}
}
public void atesleme2()
{
foreach (Game2 icin in ates2)
{
if (!icin.ruh)
{
kalanates2sayisi = kalanates2sayisi - 1;
icin.ruh = true;
icin.position = Pozisyon - icin.center;
icin.velocity = new Vector2(
(float)Math.Cos(Rota),
(float)Math.Sin(Rota)) * 20.0f - Hiz;
ses2.Play();
return;
}
}
}
void Updateyerbulucuok()
{
double enlemesine_uzaklik = (Pozisyon.X - hedefpozisyon.X);
double boylamasina_uzaklik = (Pozisyon.Y - hedefpozisyon.Y);
double Q = Math.Atan(boylamasina_uzaklik / enlemesine_uzaklik);
yerbulucuokrota = (float)Q;
double enlemesine_uzaklik2 = (Pozisyon.X - dunyapozisyon.X);
double boylamasina_uzaklik2 = (Pozisyon.Y - dunyapozisyon.Y);
double Q2 = Math.Atan(boylamasina_uzaklik2 / enlemesine_uzaklik2);
yerbulucuokrota2 = (float)Q2;
}
void UpdateGorev2ufo()
{
if (gorev2stepleri == 2)
{
#region ufo1
if (ufo1atessayim > 300)
{
ufo1atessayim = 0;
ufo1ateshiz.X = 0;
ufo1ateshiz.Y = 0;
ufo1atesrota = 0;
ufo1atespozisyon = new Vector2(-19950, -250);
}
ufo1merkez = new Vector2(gorev2ufo.Height / 2, gorev2ufo.Width / 2);
double enlemesine_uzaklik1 = (-20000 - agirliknoktasipozisyon.X);
double boylamasina_uzaklik1 = (-200 - agirliknoktasipozisyon.Y);
double Q1 = Math.Atan(boylamasina_uzaklik1 / enlemesine_uzaklik1);
ufo1atesrota = (float)Q1;
ufo1atessayim++;
ufo1atespozisyon += ufo1ateshiz;
if (Pozisyon.X > -20000)
{
ufo1ateshiz += new Vector2(
(float)Math.Cos(ufo1atesrota),
(float)Math.Sin(ufo1atesrota)) / 10f;
}
else
{
ufo1ateshiz -= new Vector2(
(float)Math.Cos(ufo1atesrota),
(float)Math.Sin(ufo1atesrota)) / 10f;
}
#endregion
#region ufo2
if (ufo2atessayim > 1000)
{
ufo2atessayim = 0;
ufo2ateshiz.X = 0;
ufo2ateshiz.Y = 0;
ufo2atesrota = 0;
ufo2atespozisyon = new Vector2(-19950, -150);
}
ufo2merkez = new Vector2(gorev2ufo.Height / 2, gorev2ufo.Width / 2);
double enlemesine_uzaklik2 = (-20000 - agirliknoktasipozisyon.X);
double boylamasina_uzaklik2 = (-100 - agirliknoktasipozisyon.Y);
double Q2 = Math.Atan(boylamasina_uzaklik2 / enlemesine_uzaklik2);
ufo2atesrota = (float)Q2;
ufo2atessayim++;
ufo2atespozisyon += ufo2ateshiz;
if (Pozisyon.X > -20000)
{
ufo2ateshiz += new Vector2(
(float)Math.Cos(ufo2atesrota),
(float)Math.Sin(ufo2atesrota)) / 10f;
}
else
{
ufo2ateshiz -= new Vector2(
(float)Math.Cos(ufo2atesrota),
(float)Math.Sin(ufo2atesrota)) / 10f;
}
#endregion
#region ufo3
if (ufo3atessayim > 100)
{
ufo3atessayim = 0;
ufo3ateshiz.X = 0;
ufo3ateshiz.Y = 0;
ufo3atesrota = 0;
ufo3atespozisyon = new Vector2(-19950, 200);
}
ufo3merkez = new Vector2(gorev2ufo.Height / 2, gorev2ufo.Width / 2);
double enlemesine_uzaklik3 = (-20000 - agirliknoktasipozisyon.X);
double boylamasina_uzaklik3 = (250 - agirliknoktasipozisyon.Y);
double Q3 = Math.Atan(boylamasina_uzaklik3 / enlemesine_uzaklik3);
ufo3atesrota = (float)Q3;
ufo3atessayim++;
ufo3atespozisyon += ufo3ateshiz;
if (Pozisyon.X > -20000)
{
ufo3ateshiz += new Vector2(
(float)Math.Cos(ufo3atesrota),
(float)Math.Sin(ufo3atesrota)) / 5f;
}
else
{
ufo3ateshiz -= new Vector2(
(float)Math.Cos(ufo3atesrota),
(float)Math.Sin(ufo3atesrota)) / 5f;
}
#endregion
#region ufo4
if (ufo4atessayim > 500)
{
ufo4atessayim = 0;
ufo4ateshiz.X = 0;
ufo4ateshiz.Y = 0;
ufo4atesrota = 0;
ufo4atespozisyon = new Vector2(-19950, 300);
}
ufo4merkez = new Vector2(gorev2ufo.Height / 2, gorev2ufo.Width / 2);
double enlemesine_uzaklik4 = (-20000 - agirliknoktasipozisyon.X);
double boylamasina_uzaklik4 = (350 - agirliknoktasipozisyon.Y);
double Q4 = Math.Atan(boylamasina_uzaklik4 / enlemesine_uzaklik4);
ufo4atesrota = (float)Q4;
ufo4atessayim++;
ufo4atespozisyon += ufo4ateshiz;
if (Pozisyon.X > -20000)
{
ufo4ateshiz += new Vector2(
(float)Math.Cos(ufo4atesrota),
(float)Math.Sin(ufo4atesrota)) / 5f;
}
else
{
ufo4ateshiz -= new Vector2(
(float)Math.Cos(ufo4atesrota),
(float)Math.Sin(ufo4atesrota)) / 5f;
}
#endregion
#region ufoates1234intersects
Rectangle aracrect = new Rectangle(
(int)agirliknoktasipozisyon.X,
(int)agirliknoktasipozisyon.Y,
agirliknoktasi.Width,
agirliknoktasi.Height);
Rectangle ufoates1rect = new Rectangle(
(int)ufo1atespozisyon.X,
(int)ufo1atespozisyon.Y,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufoates2rect = new Rectangle(
(int)ufo2atespozisyon.X,
(int)ufo2atespozisyon.Y,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufoates3rect = new Rectangle(
(int)ufo3atespozisyon.X,
(int)ufo3atespozisyon.Y,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufoates4rect = new Rectangle(
(int)ufo4atespozisyon.X,
(int)ufo4atespozisyon.Y,
gorev2ufo.Width,
gorev2ufo.Height);
if (ufoates1rect.Intersects(aracrect))
{
zirhgucu = zirhgucu - 5;
ufo1atespozisyon.X = 0;
}
if (ufoates2rect.Intersects(aracrect))
{
zirhgucu = zirhgucu - 5;
ufo2atespozisyon.X = 0;
}
if (ufoates3rect.Intersects(aracrect))
{
zirhgucu = zirhgucu - 5;
ufo3atespozisyon.X = 0;
}
if (ufoates4rect.Intersects(aracrect))
{
zirhgucu = zirhgucu - 5;
ufo4atespozisyon.X = 0;
}
#endregion
#region ufo5
if (ufo5atessayim > 300)
{
ufo5atessayim = 0;
ufo5ateshiz.X = 0;
ufo5ateshiz.Y = 0;
ufo5atesrota = 0;
ufo5atespozisyon = new Vector2(-19850, -250);
}
ufo5merkez = new Vector2(gorev2ufo.Height / 2, gorev2ufo.Width / 2);
double enlemesine_uzaklik5 = (-19850 - agirliknoktasipozisyon.X);
double boylamasina_uzaklik5 = (-250 - agirliknoktasipozisyon.Y);
double Q5 = Math.Atan(boylamasina_uzaklik5 / enlemesine_uzaklik5);
ufo5atesrota = (float)Q5;
ufo5atessayim++;
ufo5atespozisyon += ufo5ateshiz;
if (Pozisyon.X > -19850)
{
ufo5ateshiz += new Vector2(
(float)Math.Cos(ufo5atesrota),
(float)Math.Sin(ufo5atesrota)) / 15f;
}
else
{
ufo5ateshiz -= new Vector2(
(float)Math.Cos(ufo5atesrota),
(float)Math.Sin(ufo5atesrota)) / 15f;
}
#endregion
#region ufo6
if (ufo6atessayim > 300)
{
ufo6atessayim = 0;
ufo6ateshiz.X = 0;
ufo6ateshiz.Y = 0;
ufo6atesrota = 0;
ufo6atespozisyon = new Vector2(-19850, -150);
}
ufo6merkez = new Vector2(gorev2ufo.Height / 2, gorev2ufo.Width / 2);
double enlemesine_uzaklik6 = (-19850 - agirliknoktasipozisyon.X);
double boylamasina_uzaklik6 = (-150 - agirliknoktasipozisyon.Y);
double Q6 = Math.Atan(boylamasina_uzaklik6 / enlemesine_uzaklik6);
ufo6atesrota = (float)Q2;
ufo6atessayim++;
ufo6atespozisyon += ufo6ateshiz;
if (Pozisyon.X > -19850)
{
ufo6ateshiz += new Vector2(
(float)Math.Cos(ufo6atesrota),
(float)Math.Sin(ufo6atesrota)) / 15f;
}
else
{
ufo6ateshiz -= new Vector2(
(float)Math.Cos(ufo6atesrota),
(float)Math.Sin(ufo6atesrota)) / 15f;
}
#endregion
#region ufo7
if (ufo7atessayim > 300)
{
ufo7atessayim = 0;
ufo7ateshiz.X = 0;
ufo7ateshiz.Y = 0;
ufo7atesrota = 0;
ufo7atespozisyon = new Vector2(-19850, 200);
}
ufo7merkez = new Vector2(gorev2ufo.Height / 2, gorev2ufo.Width / 2);
double enlemesine_uzaklik7 = (-19850 - agirliknoktasipozisyon.X);
double boylamasina_uzaklik7 = (200 - agirliknoktasipozisyon.Y);
double Q7 = Math.Atan(boylamasina_uzaklik7 / enlemesine_uzaklik7);
ufo7atesrota = (float)Q7;
ufo7atessayim++;
ufo7atespozisyon += ufo7ateshiz;
if (Pozisyon.X > -19850)
{
ufo7ateshiz += new Vector2(
(float)Math.Cos(ufo7atesrota),
(float)Math.Sin(ufo7atesrota)) / 15f;
}
else
{
ufo7ateshiz -= new Vector2(
(float)Math.Cos(ufo7atesrota),
(float)Math.Sin(ufo7atesrota)) / 15f;
}
#endregion
#region ufo8
if (ufo8atessayim > 300)
{
ufo8atessayim = 0;
ufo8ateshiz.X = 0;
ufo8ateshiz.Y = 0;
ufo8atesrota = 0;
ufo8atespozisyon = new Vector2(-19850, 300);
}
ufo8merkez = new Vector2(gorev2ufo.Height / 2, gorev2ufo.Width / 2);
double enlemesine_uzaklik8 = (-19850 - agirliknoktasipozisyon.X);
double boylamasina_uzaklik8 = (300 - agirliknoktasipozisyon.Y);
double Q8 = Math.Atan(boylamasina_uzaklik8 / enlemesine_uzaklik8);
ufo8atesrota = (float)Q8;
ufo8atessayim++;
ufo8atespozisyon += ufo8ateshiz;
if (Pozisyon.X > -19850)
{
ufo8ateshiz += new Vector2(
(float)Math.Cos(ufo8atesrota),
(float)Math.Sin(ufo8atesrota)) / 15f;
}
else
{
ufo8ateshiz -= new Vector2(
(float)Math.Cos(ufo8atesrota),
(float)Math.Sin(ufo8atesrota)) / 15f;
}
#endregion
#region ufo9
if (ufo5atessayim > 300)
{
ufo9atessayim = 0;
ufo9ateshiz.X = 0;
ufo9ateshiz.Y = 0;
ufo9atesrota = 0;
ufo9atespozisyon = new Vector2(-19550, -250);
}
ufo9merkez = new Vector2(gorev2ufo.Height / 2, gorev2ufo.Width / 2);
double enlemesine_uzaklik9 = (-19550 - agirliknoktasipozisyon.X);
double boylamasina_uzaklik9 = (-250 - agirliknoktasipozisyon.Y);
double Q9 = Math.Atan(boylamasina_uzaklik9 / enlemesine_uzaklik9);
ufo9atesrota = (float)Q9;
ufo9atessayim++;
ufo9atespozisyon += ufo9ateshiz;
if (Pozisyon.X > -19550)
{
ufo9ateshiz += new Vector2(
(float)Math.Cos(ufo9atesrota),
(float)Math.Sin(ufo9atesrota)) / 15f;
}
else
{
ufo9ateshiz -= new Vector2(
(float)Math.Cos(ufo9atesrota),
(float)Math.Sin(ufo9atesrota)) / 15f;
}
#endregion
#region ufo10
if (ufo10atessayim > 300)
{
ufo10atessayim = 0;
ufo10ateshiz.X = 0;
ufo10ateshiz.Y = 0;
ufo10atesrota = 0;
ufo10atespozisyon = new Vector2(-19550, -150);
}
ufo10merkez = new Vector2(gorev2ufo.Height / 2, gorev2ufo.Width / 2);
double enlemesine_uzaklik10 = (-19550 - agirliknoktasipozisyon.X);
double boylamasina_uzaklik10 = (-150 - agirliknoktasipozisyon.Y);
double Q10 = Math.Atan(boylamasina_uzaklik10 / enlemesine_uzaklik10);
ufo10atesrota = (float)Q10;
ufo10atessayim++;
ufo10atespozisyon += ufo10ateshiz;
if (Pozisyon.X > -19550)
{
ufo10ateshiz += new Vector2(
(float)Math.Cos(ufo10atesrota),
(float)Math.Sin(ufo10atesrota)) / 15f;
}
else
{
ufo10ateshiz -= new Vector2(
(float)Math.Cos(ufo10atesrota),
(float)Math.Sin(ufo10atesrota)) / 15f;
}
#endregion
#region ufo11
if (ufo11atessayim > 300)
{
ufo11atessayim = 0;
ufo11ateshiz.X = 0;
ufo11ateshiz.Y = 0;
ufo11atesrota = 0;
ufo11atespozisyon = new Vector2(-19550, 200);
}
ufo11merkez = new Vector2(gorev2ufo.Height / 2, gorev2ufo.Width / 2);
double enlemesine_uzaklik11 = (-19550 - agirliknoktasipozisyon.X);
double boylamasina_uzaklik11 = (200 - agirliknoktasipozisyon.Y);
double Q11 = Math.Atan(boylamasina_uzaklik11 / enlemesine_uzaklik11);
ufo11atesrota = (float)Q11;
ufo11atessayim++;
ufo11atespozisyon += ufo11ateshiz;
if (Pozisyon.X > -19550)
{
ufo11ateshiz += new Vector2(
(float)Math.Cos(ufo11atesrota),
(float)Math.Sin(ufo11atesrota)) / 15f;
}
else
{
ufo11ateshiz -= new Vector2(
(float)Math.Cos(ufo11atesrota),
(float)Math.Sin(ufo11atesrota)) / 15f;
}
#endregion
#region ufo12
if (ufo12atessayim > 300)
{
ufo12atessayim = 0;
ufo12ateshiz.X = 0;
ufo12ateshiz.Y = 0;
ufo12atesrota = 0;
ufo12atespozisyon = new Vector2(-19550, 300);
}
ufo12merkez = new Vector2(gorev2ufo.Height / 2, gorev2ufo.Width / 2);
double enlemesine_uzaklik12 = (-19550 - agirliknoktasipozisyon.X);
double boylamasina_uzaklik12 = (300 - agirliknoktasipozisyon.Y);
double Q12 = Math.Atan(boylamasina_uzaklik12 / enlemesine_uzaklik12);
ufo12atesrota = (float)Q12;
ufo12atessayim++;
ufo12atespozisyon += ufo12ateshiz;
if (Pozisyon.X > -19550)
{
ufo12ateshiz += new Vector2(
(float)Math.Cos(ufo12atesrota),
(float)Math.Sin(ufo12atesrota)) / 15f;
}
else
{
ufo12ateshiz -= new Vector2(
(float)Math.Cos(ufo12atesrota),
(float)Math.Sin(ufo12atesrota)) / 15f;
}
#endregion
#region ufoates1234intersects
Rectangle ufoates5rect = new Rectangle(
(int)ufo5atespozisyon.X,
(int)ufo5atespozisyon.Y,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufoates6rect = new Rectangle(
(int)ufo6atespozisyon.X,
(int)ufo6atespozisyon.Y,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufoates7rect = new Rectangle(
(int)ufo7atespozisyon.X,
(int)ufo7atespozisyon.Y,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufoates8rect = new Rectangle(
(int)ufo8atespozisyon.X,
(int)ufo8atespozisyon.Y,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufoates9rect = new Rectangle(
(int)ufo9atespozisyon.X,
(int)ufo9atespozisyon.Y,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufoates10rect = new Rectangle(
(int)ufo10atespozisyon.X,
(int)ufo10atespozisyon.Y,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufoates11rect = new Rectangle(
(int)ufo11atespozisyon.X,
(int)ufo11atespozisyon.Y,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufoates12rect = new Rectangle(
(int)ufo12atespozisyon.X,
(int)ufo12atespozisyon.Y,
gorev2ufo.Width,
gorev2ufo.Height);
if (ufoates5rect.Intersects(aracrect))
{
zirhgucu = zirhgucu - 5;
ufo5atespozisyon.X = 0;
}
if (ufoates6rect.Intersects(aracrect))
{
zirhgucu = zirhgucu - 5;
ufo6atespozisyon.X = 0;
}
if (ufoates7rect.Intersects(aracrect))
{
zirhgucu = zirhgucu - 5;
ufo7atespozisyon.X = 0;
}
if (ufoates8rect.Intersects(aracrect))
{
zirhgucu = zirhgucu - 5;
ufo8atespozisyon.X = 0;
}
if (ufoates9rect.Intersects(aracrect))
{
zirhgucu = zirhgucu - 5;
ufo9atespozisyon.X = 0;
}
if (ufoates10rect.Intersects(aracrect))
{
zirhgucu = zirhgucu - 5;
ufo10atespozisyon.X = 0;
}
if (ufoates11rect.Intersects(aracrect))
{
zirhgucu = zirhgucu - 5;
ufo11atespozisyon.X = 0;
}
if (ufoates12rect.Intersects(aracrect))
{
zirhgucu = zirhgucu - 5;
ufo12atespozisyon.X = 0;
}
#endregion
}
}
void Cikti()
{
Tusabas = Keyboard.GetState();
}
private void HandleInput()
{
Tusabas = Keyboard.GetState();
}
protected override void Draw(GameTime gameTime)
{
GraphicsDevice.Clear(Color.Black);
Vector2 screenCenter = new Vector2(ScreenWidth, ScreenHeight) / 2;
Vector2 scrollOffset = screenCenter - cameraPosition;
spriteBatch.Begin();
DrawBackground(scrollOffset);
Draway(scrollOffset);
Drawdunya(scrollOffset);
Drawvenus(scrollOffset);
Drawmars(scrollOffset);
Drawates1(scrollOffset);
Drawates2(scrollOffset);
drawgoktaslari(scrollOffset);
DrawCat(scrollOffset);
spriteBatch.Draw(yanyuz, new Vector2(1089, 1), Color.White);
Drawbutonlar(scrollOffset);
DrawOverlays();
drawdonanimlar2();
drawgorev2ufo(scrollOffset);
drawUfo1234Ates(scrollOffset);
spriteBatch.Draw(yanyuz, new Vector2(1089, 1), Color.White);
Drawbutonlar(scrollOffset);
DrawOverlays();
drawdonanimlar2();
drawdeneme(scrollOffset);
drawgiris();
spriteBatch.End();
base.Draw(gameTime);
}
void drawgiris()
{
MouseState tiklagiris = Mouse.GetState();
sayim++;
if (sayim < 300)
{
spriteBatch.Draw(cntbrothers, new Vector2(0, 1), Color.White);
}
if (sayim > 300)
{
if (oyunabaslassayim < 51)
{
this.IsMouseVisible = true;
spriteBatch.Draw(menu, new Vector2(0, 1), Color.White);
spriteBatch.DrawString(yazi,
"New Game",
new Vector2(550, 300),
Color.White);
spriteBatch.DrawString(yazi,
"Credits",
new Vector2(565, 400),
Color.White);
spriteBatch.DrawString(yazi,
"Exit",
new Vector2(580, 500),
Color.White);
}
}
if (tiklagiris.LeftButton == ButtonState.Pressed && tiklagiris.X >= 552 && tiklagiris.X <= 682
&& tiklagiris.Y >= 288 && tiklagiris.Y <= 308 && oyunabaslassayim == 0)
{
oyunabasla = true;
}
if (oyunabasla == true)
{
oyunabaslassayim++;
}
if (oyunabaslassayim > 51 && oyunabaslassayim < 300)
{
spriteBatch.Draw(control, new Vector2(0, 1), Color.White);
}
if (tiklagiris.LeftButton == ButtonState.Pressed && tiklagiris.X >= 565 && tiklagiris.X <= 655
&& tiklagiris.Y >= 380 && tiklagiris.Y <= 400)
{
creditssayimcanli = true;
}
if (creditssayimcanli == true && creditsssayim != 50)
{
creditsssayim++;
}
if (creditsssayim >= 50 && creditsssayim != 0)
{
creditsssayim = 50;
spriteBatch.Draw(menu, new Vector2(0, 1), Color.White);
spriteBatch.DrawString(yazi,
"Space Game Made By Ruhi Cenet",
new Vector2(440, 300),
Color.White);
spriteBatch.DrawString(yazi,
"Back To Menu",
new Vector2(540, 400),
Color.White);
if (tiklagiris.LeftButton == ButtonState.Pressed && tiklagiris.X >= 540 && tiklagiris.X <= 705
&& tiklagiris.Y >= 380 && tiklagiris.Y <= 400)
{
creditssayimcanli = false;
}
}
if (creditssayimcanli == false && creditsssayim != 0)
{
creditsssayim--;
}
if (tiklagiris.LeftButton == ButtonState.Pressed && tiklagiris.X >= 583 && tiklagiris.X <= 620
&& tiklagiris.Y >= 475 && tiklagiris.Y <= 495)
{
this.Exit();
}
}
void DrawBackground(Vector2 scrollOffset)
{
int tileX = (int)scrollOffset.X % backgroundTexture.Width;
int tileY = (int)scrollOffset.Y % backgroundTexture.Height;
if (tileX > 0)
tileX -= backgroundTexture.Width;
if (tileY > 0)
tileY -= backgroundTexture.Height;
for (int x = tileX; x < ScreenWidth; x += backgroundTexture.Width)
{
for (int y = tileY; y < ScreenHeight; y += backgroundTexture.Height)
{
spriteBatch.Draw(backgroundTexture, new Vector2(x, y), Color.White);
}
}
}
void DrawCat(Vector2 scrollOffset)
{
agirliknoktasipozisyon.X = Pozisyon.X - 50;
agirliknoktasipozisyon.Y = Pozisyon.Y - 30;
Vector2 catCenter = new Vector2(Arac.Width, Arac.Height) / 2;
Vector2 position = Pozisyon - catCenter + scrollOffset;
spriteBatch.Draw(Arac,
position,
null,
Color.White,
Rota,
Merkez, 1f,
SpriteEffects.None, 0);
//Vector2 position2 = agirliknoktasipozisyon + scrollOffset;
//spriteBatch.Draw(agirliknoktasi, position2, Color.Yellow);
}
void DrawOverlays()
{
if (butondegiskeni == -1)
{
spriteBatch.DrawString(pozX,
"X : " + Pozisyon.X,
new Vector2(30, 30),
Color.Green);
spriteBatch.DrawString(pozY,
"Y : " + Pozisyon.Y,
new Vector2(30, 60),
Color.Green);
spriteBatch.DrawString(velocityX,
"Speed of X : " + Hiz.X,
new Vector2(30, 90),
Color.Yellow);
spriteBatch.DrawString(velocityY,
"Speed of Y : " + Hiz.Y,
new Vector2(30, 120),
Color.Yellow);
spriteBatch.DrawString(zirhgucuyazisi,
"Armour : " + zirhgucu,
new Vector2(1100, 30),
Color.White);
spriteBatch.DrawString(parayazisi,
"Money:" + para,
new Vector2(1100, 60),
Color.White);
if (ates1canlilik1 == true)
{
spriteBatch.DrawString(kalanates1yazisi,
"Weapon1:" + kalanates1sayisi,
new Vector2(1100, 90),
Color.White);
}
if (ates2canlilik2 == true)
{
spriteBatch.DrawString(kalanates2yazisi,
"Weapon2:" + kalanates2sayisi,
new Vector2(1100, 120),
Color.White);
}
spriteBatch.DrawString(yakitfont,
"Fuel : " + yakitdegeri,
new Vector2(1100, 210),
Color.White);
if (bikerealyerbulucu == 1)
{
spriteBatch.DrawString(yakitfont,
" World : ",
new Vector2(1100, 310),
Color.White);
spriteBatch.DrawString(yakitfont,
" Moon",
new Vector2(1100, 510),
Color.White);
}
if (yakitdegeri <= 0 && yakitdegeri > -100)
{
spriteBatch.DrawString(yakitfont,
"You Dont Have Any Fuel, Game Over" + yakitdegeri,
new Vector2(400, 210),
Color.White);
olum++;
if (olum > 500)
{
this.Exit();
}
}
if (zirhgucu <= 0)
{
spriteBatch.DrawString(yakitfont,
"You Died, Game Over" + yakitdegeri,
new Vector2(400, 210),
Color.White);
Hiz.X = 0;
Hiz.Y = 0;
zirhgucu = 0;
yakitdegeri = -101;
olum++;
Arac = Content.Load
if (olum > 500)
{
this.Exit();
}
}
if (gorev1zamandakika == -1)
{
spriteBatch.DrawString(yakitfont,
"Time Is Over, Game Over" + yakitdegeri,
new Vector2(400, 510),
Color.White);
Hiz.X = 0;
Hiz.Y = 0;
zirhgucu = 0;
yakitdegeri = -101;
olum++;
Arac = Content.Load
if (olum > 500)
{
this.Exit();
}
}
}
if (tikla1tikla2 == 52 && butondegiskeni == 3)
{
spriteBatch.DrawString(parayazisi,
"Money : " + para,
new Vector2(900, 120),
Color.Green);
}
}
#region gezegenler
void Draway(Vector2 scrollOffset)
{
Vector2 position = aypozisyon + scrollOffset;
spriteBatch.Draw(ay,
position,
null,
Color.White,
ayrota,
aymerkez, 1f,
SpriteEffects.None, 0);
}
void Drawdunya(Vector2 scrollOffset)
{
Vector2 position = dunyapozisyon + scrollOffset;
spriteBatch.Draw(dunya,
position,
null,
Color.White,
dunyarota,
dunyamerkez, 1f,
SpriteEffects.None, 0);
}
void Drawvenus(Vector2 scrollOffset)
{
Vector2 position = venuspozisyon + scrollOffset;
spriteBatch.Draw(venus,
position,
null,
Color.White,
venusrota,
venusmerkez, 1f,
SpriteEffects.None, 0);
}
void Drawmars(Vector2 scrollOffset)
{
Vector2 position = marspozisyon + scrollOffset;
spriteBatch.Draw(mars,
position,
null,
Color.White,
marsrota,
marsmerkez, 1f,
SpriteEffects.None, 0);
}
#endregion
#region draw atesler
void Drawates1(Vector2 scrollOffset)
{
foreach (Game2 icin in ates1)
{
if (icin.ruh == true)
{
Vector2 position = icin.position + scrollOffset;
spriteBatch.Draw(icin.nesne,
position,
null,
Color.White,
icin.rotation,
icin.center, 1f,
SpriteEffects.None, 0);
}
}
}
void Drawates2(Vector2 scrollOffset)
{
foreach (Game2 icin in ates2)
{
if (icin.ruh == true)
{
Vector2 position = icin.position + scrollOffset;
spriteBatch.Draw(icin.nesne,
position,
null,
Color.White,
icin.rotation,
icin.center, 1f,
SpriteEffects.None, 0);
}
}
}
#endregion
void Drawbutonlar(Vector2 scrollOffset)
{
MouseState tikla1 = Mouse.GetState();
MouseState tikla2 = Mouse.GetState();
Rectangle ayrect = new Rectangle(
(int)aypozisyon.X,
(int)aypozisyon.Y,
ay.Width * 1,
ay.Height * 1);
Rectangle dunyarect = new Rectangle(
(int)dunyapozisyon.X,
(int)dunyapozisyon.Y,
dunya.Width,
dunya.Height);
Rectangle aracrect = new Rectangle(
(int)Pozisyon.X - 50,
(int)Pozisyon.Y - 50,
Arac.Width,
Arac.Height);
if (aracrect.Intersects(dunyarect) && butondegiskeni == 0 || butondegiskeni == 1 || butondegiskeni == 2 || butondegiskeni == -2 || butondegiskeni == 3 || butondegiskeni == -3 || butondegiskeni == 4 || butondegiskeni == 5)
{
spriteBatch.Draw(panel1, new Vector2(0, 1), Color.White);
}
if (aracrect.Intersects(dunyarect))
{
}
else
{
this.IsMouseVisible = false;
butondegiskeni = -1;
tikla1tikla2 = -1;
}
if (aracrect.Intersects(dunyarect) && butondegiskeni == -1 && gorev1stepleri != 2 && gorev2stepleri != 2)
{
this.IsMouseVisible = true;
spriteBatch.Draw(dunyayain, new Vector2(540, 250), Color.White);
}
if (aracrect.Intersects(dunyarect) && butondegiskeni == 0)
{
//this.IsMouseVisible = true;
if (tikla1tikla2 != 25)
tikla1tikla2++;
}
//dunyayain dunyayain dunyayain dunyayain dunyayain
if (tikla1.LeftButton == ButtonState.Pressed && tikla1.X >= 540 && tikla1.X <= 690
&& tikla1.Y >= 225 && tikla1.Y <= 275 && butondegiskeni == -1)
{
butondegiskeni = 0;
Hiz.X = 0;
Hiz.Y = 0;
}
#region dunyaya indikten sonra
if (tikla1tikla2 == 25)
{
spriteBatch.Draw(goreval, new Vector2(540, 250), Color.White);
spriteBatch.Draw(tukenenler, new Vector2(540, 350), Color.White);
spriteBatch.Draw(geridon, new Vector2(540, 650), Color.White);
//gorev al gorev al gorev al gorev al gorev al gorev al
if (tikla1.LeftButton == ButtonState.Pressed && tikla1.X >= 540 && tikla1.X <= 690
&& tikla1.Y >= 235 && tikla1.Y <= 285 && butondegiskeni == 0)
{
if (gorev1stepleri == 0)
{
gorev1stepleri = 1;
butondegiskeni = -2;
}
if (gorev2stepleri == 1)
{
gorev2stepleri = 1;
butondegiskeni = -3;
}
}
//tukenenler tukenenler tukenenler tukenenler tukenenler
if (tikla1.LeftButton == ButtonState.Pressed && tikla1.X >= 540 && tikla1.X <= 690
&& tikla1.Y >= 325 && tikla1.Y <= 375 && butondegiskeni == 0)
{
butondegiskeni = 3;
}
//geridon geridon geridon geridon geridon
if (butondegiskeni == 0)
{
spriteBatch.Draw(geridon, new Vector2(540, 650), Color.White);
if (tikla2.LeftButton == ButtonState.Pressed && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 610 && tikla2.Y <= 660)
{
butondegiskeni = -1;
tikla1tikla2 = 0;
}
}
}
#endregion
#region tikla1tikla2++
if (butondegiskeni == 1 && tikla1tikla2 != 50)
{
tikla1tikla2++;
}
if (butondegiskeni == 2 && tikla1tikla2 != 49)
{
tikla1tikla2++;
}
if (butondegiskeni == -2 && tikla1tikla2 != 51)
{
tikla1tikla2++;
}
if (butondegiskeni == 3 && tikla1tikla2 != 52)
{
tikla1tikla2++;
}
if (butondegiskeni == -3 && tikla1tikla2 != 53)
{
tikla1tikla2++;
}
if (butondegiskeni == 4 && tikla1tikla2 != 54)
{
tikla1tikla2++;
}
#endregion
#region tukenenler
if (tikla1tikla2 == 52 && butondegiskeni == 3)
{
//hizgucugelistir
if (bikerealhiz == 0)
{
spriteBatch.Draw(hizgucugelistir, new Vector2(540, 50), Color.White);
if (tikla2.LeftButton == ButtonState.Released && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 40 && tikla2.Y <= 90)
{
spriteBatch.DrawString(aciklayiciyazi,
"Cost : 5000, It Makes You Faster",
new Vector2(500, 100),
Color.White);
}
if (tikla2.LeftButton == ButtonState.Pressed && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 40 && tikla2.Y <= 90 && para >= 5000)
{
hizgucu = 200;
para = para - 5000;
bikerealhiz = 1;
hizgucu = 200;
hizlanmagucu = 2.5f;
donushizi = 2.0f;
durusgucu = 10;
}
}
//zirh zirh zirh zirh zirh zirh zirh zirh
if (bikerealzirh == 0)
{
spriteBatch.Draw(zirh, new Vector2(540, 150), Color.White);
if (tikla2.LeftButton == ButtonState.Released && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 135 && tikla2.Y <= 185)
{
spriteBatch.DrawString(aciklayiciyazi,
"Cost : 1000, It Makes Your Armour 200",
new Vector2(480, 200),
Color.White);
}
if (tikla2.LeftButton == ButtonState.Pressed && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 135 && tikla2.Y <= 185 && para >= 1000)
{
para = para - 1000;
bikerealzirh = 1;
zirhgucu = 200;
}
}
//yakit yakit yakit yakit yakit yakit
if (bikerealyakit == 0)
{
spriteBatch.Draw(yakit, new Vector2(540, 250), Color.White);
if (tikla2.LeftButton == ButtonState.Released && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 235 && tikla2.Y <= 285)
{
spriteBatch.DrawString(aciklayiciyazi,
"Cost : 3000, It Makes Your Fuel 50000",
new Vector2(480, 300),
Color.White);
}
if (tikla2.LeftButton == ButtonState.Pressed && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 235 && tikla2.Y <= 285 && para >= 3000)
{
para = para - 3000;
bikerealyakit = 1;
yakitdegeri = 50000;
}
}
//silah1 silah1 silah1 silah1 silah1 silah1
if (bikerealsilah2 == 0)
{
spriteBatch.Draw(silah2, new Vector2(540, 350), Color.White);
if (tikla2.LeftButton == ButtonState.Released && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 325 && tikla2.Y <= 375)
{
spriteBatch.DrawString(aciklayiciyazi,
"Cost : 1000, It Gives Yout 500 Rocket-A" + gorev1stepleri + gorev2stepleri + butondegiskeni,
new Vector2(480, 400),
Color.White);
}
if (tikla2.LeftButton == ButtonState.Pressed && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 325 && tikla2.Y <= 375 && para >= 1000)
{
para = para - 1000;
bikerealsilah2 = 1;
ates1canlilik1 = true;
}
}
//silah2 silah2 silah2 silah2 silah2 silah2
if (bikerealsilah3 == 0)
{
spriteBatch.Draw(silah3, new Vector2(540, 450), Color.White);
if (tikla2.LeftButton == ButtonState.Released && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 425 && tikla2.Y <= 475)
{
spriteBatch.DrawString(aciklayiciyazi,
"Cost : 25000, It Gives You 50 Rocket-B",
new Vector2(480, 500),
Color.White);
}
if (tikla2.LeftButton == ButtonState.Pressed && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 425 && tikla2.Y <= 475 && para >= 25000)
{
para = para - 25000;
bikerealsilah3 = 1;
ates2canlilik2 = true;
}
}
//geridon geridon geridon geridon geridon
if (tikla1tikla2 == 52)
{
spriteBatch.Draw(geridon, new Vector2(540, 650), Color.White);
if (tikla2.LeftButton == ButtonState.Pressed && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 610 && tikla2.Y <= 660)
{
butondegiskeni = 0;
tikla1tikla2 = 0;
}
}
//yerbulucu yerbulucu yerbulucu yerbulucu yerbulucu yerbulucu
if (bikerealyerbulucu == 1)
{
spriteBatch.Draw(yerbulucual, new Vector2(540, 550), Color.White);
if (tikla2.LeftButton == ButtonState.Released && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 510 && tikla2.Y <= 560)
spriteBatch.DrawString(aciklayiciyazi,
"Cost : 99999, You Never Get Lost, It is Kind Of Helper",
new Vector2(450, 600),
Color.White);
if (tikla2.LeftButton == ButtonState.Pressed && tikla2.X >= 540 && tikla2.X <= 690
&& tikla2.Y >= 510 && tikla2.Y <= 560 && para >= 99999)
{
para = para - 99999;
this.Exit();
}
}
#endregion
}
}
void drawdeneme(Vector2 scrollOffset)
{
MouseState tikla1 = Mouse.GetState();
MouseState tikla2 = Mouse.GetState();
Rectangle ayrect = new Rectangle(
(int)aypozisyon.X,
(int)aypozisyon.Y,
ay.Width * 1,
ay.Height * 1);
Rectangle dunyarect = new Rectangle(
(int)dunyapozisyon.X,
(int)dunyapozisyon.Y,
dunya.Width,
dunya.Height);
Rectangle aracrect = new Rectangle(
(int)Pozisyon.X - 50,
(int)Pozisyon.Y - 50,
Arac.Width,
Arac.Height);
#region gorev1
if (gorev1stepleri == 1 && tikla1tikla2 == 51 && butondegiskeni == -2)
{
spriteBatch.DrawString(gorev1yazisi1,
"You Need To Drop Satellite Art1990 To Moon,",
new Vector2(100, 300),
Color.White);
spriteBatch.DrawString(gorev1yazisi2,
"If You Get Succeed, You Will Get 20.000,",
new Vector2(100, 330),
Color.White);
spriteBatch.DrawString(gorev1yazisi3,
"Your Time Is Just 1 Minute, Press The Satellite To Take Mission.",
new Vector2(100, 360),
Color.White);
spriteBatch.Draw(uydu, new Vector2(100, 390), Color.White);
if (tikla2.LeftButton == ButtonState.Pressed && tikla2.X >= 100 && tikla2.X <= 200
&& tikla2.Y >= 360 && tikla2.Y <= 460)
{
butondegiskeni = -1;
gorev1stepleri = 2;
this.IsMouseVisible = false;
}
//geridon geridon geridon geridon geridon
if (tikla1tikla2 == 51)
{
spriteBatch.Draw(geridon, new Vector2(740, 650), Color.White);
if (tikla2.LeftButton == ButtonState.Pressed && tikla2.X >= 740 && tikla2.X <= 890
&& tikla2.Y >= 610 && tikla2.Y <= 660)
{
butondegiskeni = 0;
tikla1tikla2 = 0;
gorev1stepleri = 0;
}
}
}
if (gorev1stepleri == 2)
{
spriteBatch.DrawString(gorev1zamanyazisi,
"Remining Time : " + gorev1zamandakika + "." + gorev1zamansaniye,
new Vector2(440, 30),
Color.White);
if (gorev1zamansalise != 0)
{
gorev1zamansalise = gorev1zamansalise - 1;
}
if (gorev1zamansaniye != -1 && gorev1zamansalise == 0)
{
gorev1zamansalise = 100;
gorev1zamansaniye = gorev1zamansaniye - 1;
}
if (gorev1zamansaniye == -1 && gorev1zamandakika != 0)
{
gorev1zamansaniye = 59;
gorev1zamandakika = gorev1zamandakika - 1;
}
if (gorev1zamansaniye == -1)
{
gorev1zamansaniye = 0;
}
if (aracrect.Intersects(ayrect))
{
if (Hiz.X > 5 || Hiz.Y > 5 || Hiz.X < -5 || Hiz.Y < -5)
{
spriteBatch.DrawString(gorev1zamanyazisi,
"You Are Too Fast To Drop Satellite",
new Vector2(350, 60),
Color.White);
}
if (Hiz.X < 5 && Hiz.Y < 5 && Hiz.X > -5 && Hiz.Y > -5)
{
spriteBatch.DrawString(gorev1zamanyazisi,
"Press Enter To Drop Satellite",
new Vector2(350, 60),
Color.White);
if (Tusabas.IsKeyDown(Keys.Enter))
{
gorev1stepleri = 3;
gorev2stepleri = 1;
}
}
}
}
if (gorev1stepleri == 3 && gorev1bitiris != 300 && gorev1bitiris != -1)
{
gorev1bitiris++;
spriteBatch.DrawString(gorev1zamanyazisi,
"Congratulations, Mission Completed, 20.000",
new Vector2(350, 60),
Color.White);
para = para + 100;
}
if (gorev1bitiris == 500 && gorev1stepleri == 3)
{
gorev2stepleri = 1;
gorev1stepleri = 4;
gorev1bitiris = -1;
}
#endregion
#region gorev2
if (gorev2stepleri == 1 && tikla1tikla2 == 53 && butondegiskeni == -3)
{
spriteBatch.DrawString(gorev1yazisi1,
"We Are Getting Bad Signals From Art 1990,",
new Vector2(100, 300),
Color.White);
spriteBatch.DrawString(gorev1yazisi2,
"You Need To Solve Our Problem, If You Can, You Will Collect 100000,",
new Vector2(100, 330),
Color.White);
spriteBatch.DrawString(gorev1yazisi3,
"Your Time is 15 Minutes, Press The Moon To Take Mission.",
new Vector2(100, 360),
Color.White);
spriteBatch.Draw(ay, new Vector2(100, 390), Color.White);
if (tikla2.LeftButton == ButtonState.Pressed && tikla2.X >= 100 && tikla2.X <= 300
&& tikla2.Y >= 360 && tikla2.Y <= 550)
{
butondegiskeni = -1;
gorev2stepleri = 2;
this.IsMouseVisible = false;
}
//geridon geridon geridon geridon geridon
if (tikla1tikla2 == 53)
{
spriteBatch.Draw(geridon, new Vector2(740, 650), Color.White);
if (tikla2.LeftButton == ButtonState.Pressed && tikla2.X >= 740 && tikla2.X <= 890
&& tikla2.Y >= 610 && tikla2.Y <= 660)
{
butondegiskeni = 0;
tikla1tikla2 = 0;
}
}
gorev1zamandakika = 15;
gorev1zamansaniye = 00;
gorev1zamansalise = 100;
}
if (gorev2stepleri == 2)
{
if (gorev1zamansalise != 0)
{
gorev1zamansalise = gorev1zamansalise - 1;
}
if (gorev1zamansaniye != -1 && gorev1zamansalise == 0)
{
gorev1zamansalise = 100;
gorev1zamansaniye = gorev1zamansaniye - 1;
}
if (gorev1zamansaniye == -1 && gorev1zamandakika != 0)
{
gorev1zamansaniye = 59;
gorev1zamandakika = gorev1zamandakika - 1;
}
if (gorev1zamansaniye == -1)
{
gorev1zamansaniye = 0;
}
}
if (gorev1bitiris != 1000 && gorev2stepleri == 3)
{
gorev1bitiris++;
spriteBatch.DrawString(gorev1zamanyazisi,
"Congratulations, Mission Completed, 100.100",
new Vector2(350, 60),
Color.White);
para = para + 100;
}
if (gorev1bitiris == 1500 && gorev2stepleri == 3)
{
gorev2stepleri = 4;
gorev1bitiris = -2;
}
#endregion
}
void drawgorev2ufo(Vector2 scrollOffset)
{
if (gorev2stepleri == 2)
{
if (ufo1can > 0)
{
Vector2 position1 = new Vector2(-20000, -200) + scrollOffset;
spriteBatch.Draw(gorev2ufo,
position1,
null,
Color.White,
ufo1rota,
ufo1merkez, 1f,
SpriteEffects.None, 0);
gorev2agirliknoktasipozisyonu = new Vector2(-19950, -250);
Vector2 position1agirliknoktasi = gorev2agirliknoktasipozisyonu + scrollOffset;
spriteBatch.Draw(gorev2agirliknoktasi, position1agirliknoktasi, Color.Yellow);
}
if (ufo2can > 0)
{
Vector2 position2 = new Vector2(-20000, -100) + scrollOffset;
spriteBatch.Draw(gorev2ufo,
position2,
null,
Color.White,
ufo2rota,
ufo2merkez, 1f,
SpriteEffects.None, 0);
gorev2agirliknoktasipozisyonu = new Vector2(-19950, -150);
Vector2 position2agirliknoktasi = gorev2agirliknoktasipozisyonu + scrollOffset;
spriteBatch.Draw(gorev2agirliknoktasi, position2agirliknoktasi, Color.Yellow);
}
if (ufo3can > 0)
{
Vector2 position3 = new Vector2(-20000, 250) + scrollOffset;
spriteBatch.Draw(gorev2ufo,
position3,
null,
Color.White,
ufo3rota,
ufo3merkez, 1f,
SpriteEffects.None, 0);
gorev2agirliknoktasipozisyonu = new Vector2(-19950, 200);
Vector2 position3agirliknoktasi = gorev2agirliknoktasipozisyonu + scrollOffset;
spriteBatch.Draw(gorev2agirliknoktasi, position3agirliknoktasi, Color.Yellow);
}
if (ufo4can > 0)
{
Vector2 position4 = new Vector2(-20000, 350) + scrollOffset;
spriteBatch.Draw(gorev2ufo,
position4,
null,
Color.White,
ufo4rota,
ufo4merkez, 1f,
SpriteEffects.None, 0);
gorev2agirliknoktasipozisyonu = new Vector2(-19950, 300);
Vector2 position4agirliknoktasi = gorev2agirliknoktasipozisyonu + scrollOffset;
spriteBatch.Draw(gorev2agirliknoktasi, position4agirliknoktasi, Color.Yellow);
}
if (ufo5can > 0)
{
Vector2 position1 = new Vector2(-19850, -200) + scrollOffset;
spriteBatch.Draw(gorev2ufo,
position1,
null,
Color.White,
ufo5rota,
ufo5merkez, 1f,
SpriteEffects.None, 0);
gorev2agirliknoktasipozisyonu = new Vector2(-19800, -250);
Vector2 position1agirliknoktasi = gorev2agirliknoktasipozisyonu + scrollOffset;
spriteBatch.Draw(gorev2agirliknoktasi, position1agirliknoktasi, Color.Yellow);
}
if (ufo6can > 0)
{
Vector2 position2 = new Vector2(-19850, -100) + scrollOffset;
spriteBatch.Draw(gorev2ufo,
position2,
null,
Color.White,
ufo6rota,
ufo6merkez, 1f,
SpriteEffects.None, 0);
gorev2agirliknoktasipozisyonu = new Vector2(-19800, -150);
Vector2 position2agirliknoktasi = gorev2agirliknoktasipozisyonu + scrollOffset;
spriteBatch.Draw(gorev2agirliknoktasi, position2agirliknoktasi, Color.Yellow);
}
if (ufo7can > 0)
{
Vector2 position3 = new Vector2(-19850, 250) + scrollOffset;
spriteBatch.Draw(gorev2ufo,
position3,
null,
Color.White,
ufo7rota,
ufo7merkez, 1f,
SpriteEffects.None, 0);
gorev2agirliknoktasipozisyonu = new Vector2(-19800, 200);
Vector2 position3agirliknoktasi = gorev2agirliknoktasipozisyonu + scrollOffset;
spriteBatch.Draw(gorev2agirliknoktasi, position3agirliknoktasi, Color.Yellow);
}
if (ufo8can > 0)
{
Vector2 position4 = new Vector2(-19850, 350) + scrollOffset;
spriteBatch.Draw(gorev2ufo,
position4,
null,
Color.White,
ufo8rota,
ufo8merkez, 1f,
SpriteEffects.None, 0);
gorev2agirliknoktasipozisyonu = new Vector2(-19800, 300);
Vector2 position4agirliknoktasi = gorev2agirliknoktasipozisyonu + scrollOffset;
spriteBatch.Draw(gorev2agirliknoktasi, position4agirliknoktasi, Color.Yellow);
}
if (ufo9can > 0)
{
Vector2 position5 = new Vector2(-19550, -200) + scrollOffset;
spriteBatch.Draw(gorev2ufo,
position5,
null,
Color.White,
ufo9rota,
ufo9merkez, 1f,
SpriteEffects.None, 0);
gorev2agirliknoktasipozisyonu = new Vector2(-19500, -250);
Vector2 position5agirliknoktasi = gorev2agirliknoktasipozisyonu + scrollOffset;
spriteBatch.Draw(gorev2agirliknoktasi, position5agirliknoktasi, Color.Yellow);
}
if (ufo10can > 0)
{
Vector2 position6 = new Vector2(-19550, -100) + scrollOffset;
spriteBatch.Draw(gorev2ufo,
position6,
null,
Color.White,
ufo10rota,
ufo10merkez, 1f,
SpriteEffects.None, 0);
gorev2agirliknoktasipozisyonu = new Vector2(-19500, -150);
Vector2 position6agirliknoktasi = gorev2agirliknoktasipozisyonu + scrollOffset;
spriteBatch.Draw(gorev2agirliknoktasi, position6agirliknoktasi, Color.Yellow);
}
if (ufo11can > 0)
{
Vector2 position7 = new Vector2(-19550, 250) + scrollOffset;
spriteBatch.Draw(gorev2ufo,
position7,
null,
Color.White,
ufo11rota,
ufo11merkez, 1f,
SpriteEffects.None, 0);
gorev2agirliknoktasipozisyonu = new Vector2(-19500, 200);
Vector2 position7agirliknoktasi = gorev2agirliknoktasipozisyonu + scrollOffset;
spriteBatch.Draw(gorev2agirliknoktasi, position7agirliknoktasi, Color.Yellow);
}
if (ufo12can > 0)
{
Vector2 position8 = new Vector2(-19550, 350) + scrollOffset;
spriteBatch.Draw(gorev2ufo,
position8,
null,
Color.White,
ufo12rota,
ufo12merkez, 1f,
SpriteEffects.None, 0);
gorev2agirliknoktasipozisyonu = new Vector2(-19500, 300);
Vector2 position8agirliknoktasi = gorev2agirliknoktasipozisyonu + scrollOffset;
spriteBatch.Draw(gorev2agirliknoktasi, position8agirliknoktasi, Color.Yellow);
}
foreach (Game2 icin in ates1)
{
#region intersects
Rectangle ates1rect = new Rectangle(
(int)icin.position.X,
(int)icin.position.Y,
icin.nesne.Width,
icin.nesne.Height);
Rectangle ufo1rect = new Rectangle(
(int)-20000,
(int)-270,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo2rect = new Rectangle(
(int)-20000,
(int)-170,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo3rect = new Rectangle(
(int)-20000,
(int)200,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo4rect = new Rectangle(
(int)-20000,
(int)300,
gorev2ufo.Width,
gorev2ufo.Height);
if (ates1rect.Intersects(ufo1rect) && ufo1can > 0)
{
ufo1can = ufo1can - 5;
icin.ruh = false;
}
if (ates1rect.Intersects(ufo2rect) && ufo2can > 0)
{
ufo2can = ufo2can - 5;
icin.ruh = false;
}
if (ates1rect.Intersects(ufo3rect) && ufo3can > 0)
{
ufo3can = ufo3can - 5;
icin.ruh = false;
}
if (ates1rect.Intersects(ufo4rect) && ufo4can > 0)
{
ufo4can = ufo4can - 5;
icin.ruh = false;
}
Rectangle ufo5rect = new Rectangle(
(int)-19850,
(int)-270,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo6rect = new Rectangle(
(int)-19850,
(int)-170,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo7rect = new Rectangle(
(int)-19850,
(int)200,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo8rect = new Rectangle(
(int)-19850,
(int)300,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo9rect = new Rectangle(
(int)-19550,
(int)-270,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo10rect = new Rectangle(
(int)-19550,
(int)-170,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo11rect = new Rectangle(
(int)-19550,
(int)200,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo12rect = new Rectangle(
(int)-19550,
(int)300,
gorev2ufo.Width,
gorev2ufo.Height);
if (ates1rect.Intersects(ufo5rect) && ufo5can > 0)
{
ufo5can = ufo5can - 5;
icin.ruh = false;
}
if (ates1rect.Intersects(ufo6rect) && ufo6can > 0)
{
ufo6can = ufo6can - 5;
icin.ruh = false;
}
if (ates1rect.Intersects(ufo7rect) && ufo7can > 0)
{
ufo7can = ufo7can - 5;
icin.ruh = false;
}
if (ates1rect.Intersects(ufo8rect) && ufo8can > 0)
{
ufo8can = ufo8can - 5;
icin.ruh = false;
}
if (ates1rect.Intersects(ufo9rect) && ufo9can > 0)
{
ufo9can = ufo9can - 5;
icin.ruh = false;
}
if (ates1rect.Intersects(ufo10rect) && ufo10can > 0)
{
ufo10can = ufo10can - 5;
icin.ruh = false;
}
if (ates1rect.Intersects(ufo11rect) && ufo11can > 0)
{
ufo11can = ufo11can - 5;
icin.ruh = false;
}
if (ates1rect.Intersects(ufo12rect) && ufo12can > 0)
{
ufo12can = ufo12can - 5;
icin.ruh = false;
}
#endregion
}
foreach (Game2 icin in ates2)
{
#region intersects
Rectangle ates2rect = new Rectangle(
(int)icin.position.X,
(int)icin.position.Y,
icin.nesne.Width,
icin.nesne.Height);
Rectangle ufo1rect = new Rectangle(
(int)-20000,
(int)-270,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo2rect = new Rectangle(
(int)-20000,
(int)-170,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo3rect = new Rectangle(
(int)-20000,
(int)200,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo4rect = new Rectangle(
(int)-20000,
(int)300,
gorev2ufo.Width,
gorev2ufo.Height);
if (ates2rect.Intersects(ufo1rect) && ufo1can > 0)
{
ufo1can = ufo1can - 25;
icin.ruh = false;
}
if (ates2rect.Intersects(ufo2rect) && ufo2can > 0)
{
ufo2can = ufo2can - 25;
icin.ruh = false;
}
if (ates2rect.Intersects(ufo3rect) && ufo3can > 0)
{
ufo3can = ufo3can - 25;
icin.ruh = false;
}
if (ates2rect.Intersects(ufo4rect) && ufo4can > 0)
{
ufo4can = ufo4can - 25;
icin.ruh = false;
}
Rectangle ufo5rect = new Rectangle(
(int)-19850,
(int)-270,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo6rect = new Rectangle(
(int)-19850,
(int)-170,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo7rect = new Rectangle(
(int)-19850,
(int)200,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo8rect = new Rectangle(
(int)-19850,
(int)300,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo9rect = new Rectangle(
(int)-19550,
(int)-270,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo10rect = new Rectangle(
(int)-19550,
(int)-170,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo11rect = new Rectangle(
(int)-19550,
(int)200,
gorev2ufo.Width,
gorev2ufo.Height);
Rectangle ufo12rect = new Rectangle(
(int)-19550,
(int)300,
gorev2ufo.Width,
gorev2ufo.Height);
if (ates2rect.Intersects(ufo5rect) && ufo5can > 0)
{
ufo5can = ufo5can - 25;
icin.ruh = false;
}
if (ates2rect.Intersects(ufo6rect) && ufo6can > 0)
{
ufo6can = ufo6can - 25;
icin.ruh = false;
}
if (ates2rect.Intersects(ufo7rect) && ufo7can > 0)
{
ufo7can = ufo7can - 25;
icin.ruh = false;
}
if (ates2rect.Intersects(ufo8rect) && ufo8can > 0)
{
ufo8can = ufo8can - 25;
icin.ruh = false;
}
if (ates2rect.Intersects(ufo9rect) && ufo9can > 0)
{
ufo9can = ufo9can - 25;
icin.ruh = false;
}
if (ates2rect.Intersects(ufo10rect) && ufo10can > 0)
{
ufo10can = ufo10can - 25;
icin.ruh = false;
}
if (ates2rect.Intersects(ufo11rect) && ufo11can > 0)
{
ufo11can = ufo11can - 25;
icin.ruh = false;
}
if (ates2rect.Intersects(ufo12rect) && ufo12can > 0)
{
ufo12can = ufo12can - 25;
icin.ruh = false;
}
#endregion
}
spriteBatch.DrawString(gorev1zamanyazisi,
"Remining Time : " + gorev1zamandakika + "." + gorev1zamansaniye,
new Vector2(440, 30),
Color.White);
if (Pozisyon.X < -18000 && Pozisyon.X > -22000 && Pozisyon.Y > -2000 && Pozisyon.Y < 2000)
{
spriteBatch.DrawString(gorev1zamanyazisi,
"Kill Them All",
new Vector2(440, 60),
Color.White);
}
if (ufo1can <= 0 && ufo2can <= 0 && ufo3can <= 0 && ufo4can <= 0 && ufo5can <= 0 && ufo6can <= 0 && ufo7can <= 0 && ufo9can <= 0 && ufo10can <= 0 && ufo11can <= 0 && ufo12can <= 0 && ufo8can <= 0)
{
gorev2stepleri = 3;
}
}
}
void drawUfo1234Ates(Vector2 scrollOffset)
{
if (gorev2stepleri == 2 && ufo1can > 0)
{
Vector2 position1 = ufo1atespozisyon + scrollOffset;
spriteBatch.Draw(ufo1ates, position1, Color.White);
}
if (gorev2stepleri == 2 && ufo2can > 0)
{
Vector2 position2 = ufo2atespozisyon + scrollOffset;
spriteBatch.Draw(ufo1ates, position2, Color.White);
}
if (gorev2stepleri == 2 && ufo3can > 0)
{
Vector2 position3 = ufo3atespozisyon + scrollOffset;
spriteBatch.Draw(ufo1ates, position3, Color.White);
}
if (gorev2stepleri == 2 && ufo4can > 0)
{
Vector2 position4 = ufo4atespozisyon + scrollOffset;
spriteBatch.Draw(ufo1ates, position4, Color.White);
}
if (gorev2stepleri == 2 && ufo5can > 0)
{
Vector2 position1 = ufo5atespozisyon + scrollOffset;
spriteBatch.Draw(ufo5ates, position1, Color.White);
}
if (gorev2stepleri == 2 && ufo6can > 0)
{
Vector2 position2 = ufo6atespozisyon + scrollOffset;
spriteBatch.Draw(ufo6ates, position2, Color.White);
}
if (gorev2stepleri == 2 && ufo7can > 0)
{
Vector2 position3 = ufo7atespozisyon + scrollOffset;
spriteBatch.Draw(ufo7ates, position3, Color.White);
}
if (gorev2stepleri == 2 && ufo8can > 0)
{
Vector2 position4 = ufo8atespozisyon + scrollOffset;
spriteBatch.Draw(ufo8ates, position4, Color.White);
}
if (gorev2stepleri == 2 && ufo9can > 0)
{
Vector2 position5 = ufo9atespozisyon + scrollOffset;
spriteBatch.Draw(ufo9ates, position5, Color.White);
}
if (gorev2stepleri == 2 && ufo10can > 0)
{
Vector2 position6 = ufo10atespozisyon + scrollOffset;
spriteBatch.Draw(ufo10ates, position6, Color.White);
}
if (gorev2stepleri == 2 && ufo11can > 0)
{
Vector2 position7 = ufo11atespozisyon + scrollOffset;
spriteBatch.Draw(ufo11ates, position7, Color.White);
}
if (gorev2stepleri == 2 && ufo12can > 0)
{
Vector2 position8 = ufo12atespozisyon + scrollOffset;
spriteBatch.Draw(ufo12ates, position8, Color.White);
}
}
void drawdonanimlar2()
{
if (bikerealyerbulucu == 1 && butondegiskeni == -1)
{
if (hedefpozisyon.X > Pozisyon.X)
{
spriteBatch.Draw(yerbulucuok2, new Vector2(1185, 600), null, Color.White,
yerbulucuokrota, yerbulucuokmerkez, 2f, SpriteEffects.None, 0);
}
else
{
spriteBatch.Draw(yerbulucuok1, new Vector2(1185, 600), null, Color.White,
yerbulucuokrota, yerbulucuokmerkez, 2f, SpriteEffects.None, 0);
}
hedefpozisyon = aypozisyon;
if (bikerealyerbulucu == 1 && butondegiskeni == -1)
{
if (dunyapozisyon.X > Pozisyon.X)
{
spriteBatch.Draw(yerbulucuok2, new Vector2(1185, 400), null, Color.White,
yerbulucuokrota2, yerbulucuokmerkez, 2f, SpriteEffects.None, 0);
}
else
{
spriteBatch.Draw(yerbulucuok1, new Vector2(1185, 400), null, Color.White,
yerbulucuokrota2, yerbulucuokmerkez, 2f, SpriteEffects.None, 0);
}
hedefpozisyon = aypozisyon;
}
}
}
void drawgoktaslari(Vector2 scrollOfset)
{
Vector2 position1 = goktasi1pozisyon + scrollOfset;
spriteBatch.Draw(goktasi1, position1, null, Color.White,
goktasi1rota, goktasi1merkez, 1f, SpriteEffects.None, 0);
Vector2 position2 = goktasi2pozisyon + scrollOfset;
spriteBatch.Draw(goktasi2, position2, null, Color.White,
goktasi2rota, goktasi2merkez, 1f, SpriteEffects.None, 0);
Vector2 position3 = goktasi3pozisyon + scrollOfset;
spriteBatch.Draw(goktasi3, position3, null, Color.White,
goktasi3rota, goktasi3merkez, 1f, SpriteEffects.None, 0);
Vector2 position4 = goktasi4pozisyon + scrollOfset;
spriteBatch.Draw(goktasi4, position4, null, Color.White,
goktasi4rota, goktasi4merkez, 1f, SpriteEffects.None, 0);
}
}
}
GAME 2
using System;
using System.Collections.Generic;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Audio;
using Microsoft.Xna.Framework.Content;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Input;
using Microsoft.Xna.Framework.Storage;
namespace Space_Game
{
class Game2
{
public Texture2D nesne;
public Vector2 position;
public float rotation;
public Vector2 center;
public Vector2 velocity;
public bool ruh;
public Vector2 boyut;
public Game2(Texture2D nesneolusturma)
{
rotation = 0.0f;
position = new Vector2(600, 600);
nesne = nesneolusturma;
center = new Vector2(nesne.Width / 2, nesne.Height / 2);
velocity = Vector2.Zero;
ruh = false;
}
}
}