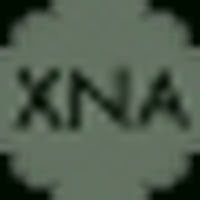
You can think our first game is too easy,
but this is the beginning that i should tell.
We will create a ball, and we will control it by keyboard.
Texture2D top; (top is the name of our object, and its a variable, if you want to chagne it, you can)
We should write a position for our object, i mean position of top.
Vector2 pozisyon = new Vector2(400, 300);
Vector2 hiz; (speed of top)
float rota; (rotate of top)
Vector2 merkez; (center of top)
And we should load picture of top.
top = Content.Load
Updating
KeyboardState tusabas = Keyboard.GetState(); (tusabas is also a variable)
pozisyon += hiz;
int MaxX = graphics.GraphicsDevice.Viewport.Width - top.Width;
int MinX = 0;
int MaxY = graphics.GraphicsDevice.Viewport.Height - top.Height;
int MinY = 0;
if (tusabas.IsKeyDown(Keys.Escape))
{
this.Exit();
}
if (tusabas.IsKeyDown(Keys.Up))
{
hiz.Y -= 0.5f;
}
if (tusabas.IsKeyDown(Keys.Down))
{
hiz.Y += 0.5f;
}
if (tusabas.IsKeyDown(Keys.Left))
{
hiz.X -= 0.5f;
}
if (tusabas.IsKeyDown(Keys.Right))
{
hiz.X += 0.5f;
}
if (pozisyon.X < MinX)
{
pozisyon.X = pozisyon.X + 1;
hiz.X = -hiz.X;
}
if (pozisyon.X > MaxX)
{
pozisyon.X = pozisyon.X - 1;
hiz.X = -hiz.X;
}
if (pozisyon.Y < MinY)
{
pozisyon.Y = pozisyon.Y + 1;
hiz.Y = -hiz.Y;
}
if (pozisyon.Y > MaxY)
{
pozisyon.Y = pozisyon.Y - 1;
hiz.Y = -hiz.Y;
}
if (hiz.X > 15)
{
hiz.X = 15;
}
if (hiz.X < -15)
{
hiz.X = -15;
}
if (hiz.Y > 15)
{
hiz.Y = 15;
}
if (hiz.Y < -15)
{
hiz.Y = -15;
}
Drawing
spriteBatch.Begin(SpriteBlendMode.AlphaBlend);
spriteBatch.Draw(top, pozisyon, null, Color.White, rota, merkez, 1f, SpriteEffects.None, 0f);
spriteBatch.End();
Thats it, our first game is ready...
This is the link of what we just programmed.